Heap Sort
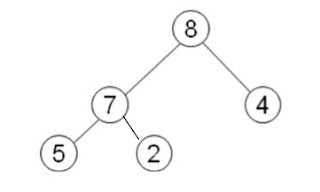
Heap Sort A heap is a nearly complete binary tree with the following two properties: Structural property: all levels are full, except possibly the last one, which is filled from left to right Order (heap) property: for any node x, Parent(x) ≥ x Array Representation of Heaps A heap can be stored as an array A. Root of tree is A[1] Left child of A[i] = A[2i] Right child of A[i] = A[2i + 1] Parent of A[i] = A[ i/2 ] Heapsize[A] ≤ length[A] The elements in the subarray A[( ⌊ n/2 ⌋ +1) .. n] are leaves Max-heaps (largest element at root), have the max-heap property: for all nodes i, excluding the root: A[PARENT(i)] ≥ A[i] Min-heaps (smallest element at root), have the min-heap property: for all nodes i, excluding the root: A[PARENT(i)] ≤ A[i] Adding/Deleting Nodes New nodes are always inserted at the bottom level (left to right) and nodes are removed from the bottom level (right to left). Operations on Heaps Maintai