Data Representation in Computer
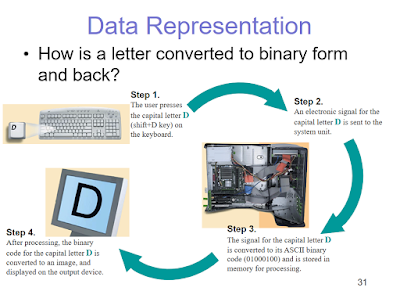
How Computers Represent Data All symbols, pictures, or words must be reduced to a string of binary digits. A binary digit is called a bit and represents either a 0 or a 1. These are the only digits in the binary or base 2, number system used by computers. A string of eight bits used to store one number or character in a computer system is called a byte. One byte for character A 01000001 The computer representation in ASCII for the name Alice is 01000001 = A 01001100 = L 01001001 = I 01000011 = C 01000101 = E To represent the numbers 0 through 9 and the letters a through z and A through Z , computer designers have created coding systems consisting of several hundred standard codes. In one code, for instance, the binary number 01000001 stands for the letter A . Two common coding systems are Extended Binary Coded Decimal Interchange Code (EBCDIC) and American Standard Code for Information Interchange (ASCII). EBCDIC represents every number, alphabetic character, or spe