Insertion Sort
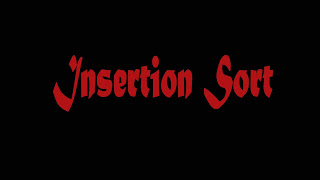
Insertion Sort An insertion sort is one that sorts a set of values by inserting values into an existing sorted file. Suppose that Iist[] is a list of n elements in memory. The insertion sort technique scans the list Iist[] from Iist[1] to list[n-1], inserting each element list[ k] into its proper position in the previously sorted sublist list[o], list[1], ..., list[n-1]. Illustration: Let list[] be an array of 7 elements: 25, 15, 30, 9, 99, 20, 26. Initial scenario, Step 1: list[1] Step 2: list[2]>list[1] , so position of elements remain same. Step 3: list[3] is less than list[2.],list [1] and list[o], thus list[3] is inserted at list[o] position and others are shifted by one position. Step 4: list[4]>list[3] , so position of elements remain same. Step 5: list[5] is less than list[4], list[3], and list[2], so list[5] is inserted at the position of list[2] and others are shifted by one position. Step 6: list