Escape Sequence in C Programming | C Programming
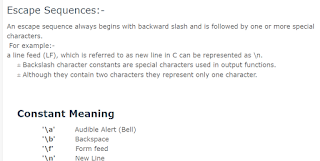
Escape Sequences in C Programming:- An escape sequence always begins with backward slash and is followed by one or more special characters. For example:- a line feed (LF), which is referred to as new line in C can be represented as \n. Backslash character constants are special characters used in output functions. Although they contain two characters they represent only one character.