C Program For Prim's Algorithm To Find Shortest Path | C Programming
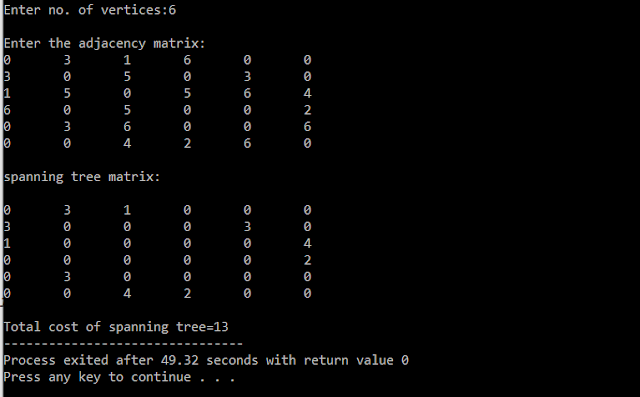
C Program For Prim's Algorithm To Find Shortest Path #include <stdio.h> #include <stdlib.h> #define infinity 9999 #define MAX 20 int G [ MAX ][ MAX ], spanning [ MAX ][ MAX ], n ; int prims (); int main () { int i , j , total_cost ; printf ( "Enter no. of vertices:" ); scanf ( " %d " , & n ); printf ( " \n Enter the adjacency matrix: \n " ); for ( i = 0 ; i < n ; i ++) for ( j = 0 ; j < n ; j ++) scanf ( " %d " , & G [ i ][ j ]); total_cost = prims (); printf ( " \n spanning tree matrix: \n " ); for ( i = 0 ; i < n ; i ++) { printf ( " \n " ); for ( j = 0 ; j < n ; j ++) printf ( " %d \t " , spanning [ i ][ j ]); } printf ( " \n\n Total cost of spanning tree= %d " , total_cost ); return 0 ; } int prims () { int cost [ MAX ][ MAX ]; int u ,